Here is homework for cs-110. Is there any problem for it? If not, what's the whole source code?
Description Write a program that will convert a distance entered in inches to a distance in yards, feet and inches. The program will ask the user to i
Description
Write a program that will convert a distance entered in inches to a distance in yards, feet and inches.
The program will ask the user to input a distance in inches as a whole number. It will convert the distance entered into yards, feet and inches. Then it will display the distance in yards, feet and inches. See the Test Data section below.
For example, the program will convert 5o inches into 1 yard, 1 ft and 2 inches.
Test Data
Test Run
(User input is shown in bolds).
Enter distance in inches:
140
Yards: 3
Feet: 2
Inches: 8
Details
Implementation
Sample Code
int inches, feet, yards;
inches = 50;
// Convert inches into feet and inches.
// The quotient below will return the feet.
feet = inches/12; //This computes quotient. So feet is now 4. Remainder is ignored.
//The remainder below will return the inches.
inches = inches%12; //This compute the remainder. So inches is now 2. Quotient is ignored.
//Conver feet into yards and feet.
//Repeat the above process.
//Find yards (from feet) by using quotient operator ( / ).
yards = feet / 3; //yards is now 1
//Find the remaining feet by using remainder operator (%).
feet = feet % 3; //feet is now 1
Discussion
There is a difference between decimal division and integer division.
They both use the same symbol “/ “.
But the meaning of the symbol “/ “ is different depending upon the context it is used in.
Decimal Division:
If one or both the operands around the division symbol are decimal variables or constants, a decimal division is performed.
In a decimal division, the answer is a decimal number. The remainder is irrelevant.
Integer Division:
If both the operands around the division symbol are whole number variables or constants, an integer division is done.
In an integer division, the whole number quotient is the answer, the remainder is ignored.
To find remainder in an integer division, use the remainder operator ( % ).
The remainder operator works only when both the operands are whole number variables or constants.
Examples:
14/4; //Integer division. Answer 3.
14/4.0; //Decimal division. Answer 3.5
14.0/4.0; //Decimal division. Answer 3.5
14 % 4; //Remainder operator. Answer 2
14 % 4.0; //Illegal. Remainder operator not available for decimal values.
14.0 % 4; //Illegal
double d = 14/4.0; //d is 3.5
double d = 14.0/4.0; //d is 3.5
double d2 = 14/4; //d2 is 3
int i = 14/4; //i is 3
double x = 14;
double z = x / 4; //z is 3.5
or:Description Write a program that will convert a distance entered in inches to a distance in yards, feet and inches. The program will ask the user to input a distance in inches as a whole number. It will convert the distance entered into yards, feet and inches. Then it will display the distance in yards, feet and inches. See the Test Data section below. For example, the program will convert 5o inches into 1 yard, 1 ft and 2 inches. Test Data Test Run(User input is shown in bolds). Enter distance in inches:140 Yards: 3Feet: 2Inches: 8 Details Implementation Sample Code int inches, feet, yards; inches = 50; // Convert inches into feet and inches. // The quotient below will return the feet.feet = inches/12; //This computes quotient. So feet is now 4. Remainder is ignored. //The remainder below will return the inches.inches = inches%12; //This compute the remainder. So inches is now 2. Quotient is ignored. //Conver feet into yards and feet. //Repeat the above process.//Find yards (from feet) by using quotient operator ( / ).yards = feet / 3; //yards is now 1 //Find the remaining feet by using remainder operator (%).feet = feet % 3; //feet is now 1 Discussion There is a difference between decimal division and integer division.They both use the same symbol \u201c/ \u201c.But the meaning of the symbol \u201c/ \u201c is different depending upon the context it is used in. Decimal Division:If one or both the operands around the division symbol are decimal variables or constants, a decimal division is performed.In a decimal division, the answer is a decimal number. The remainder is irrelevant. Integer Division:If both the operands around the division symbol are whole number variables or constants, an integer division is done.In an integer division, the whole number quotient is the answer, the remainder is ignored.To find remainder in an integer division, use the remainder operator ( % ).The remainder operator works only when both the operands are whole number variables or constants. Examples: 14/4; //Integer division. Answer 3. 14/4.0; //Decimal division. Answer 3.5 14.0/4.0; //Decimal division. Answer 3.5 14 % 4; //Remainder operator. Answer 2 14 % 4.0; //Illegal. Remainder operator not available for decimal values.14.0 % 4; //Illegal double d = 14/4.0; //d is 3.5double d = 14.0/4.0; //d is 3.5double d2 = 14/4; //d2 is 3int i = 14/4; //i is 3 double x = 14;double z = x / 4; //z is 3.5
or:there may be a problem with java. ask in the ICT topic
Tags:code,
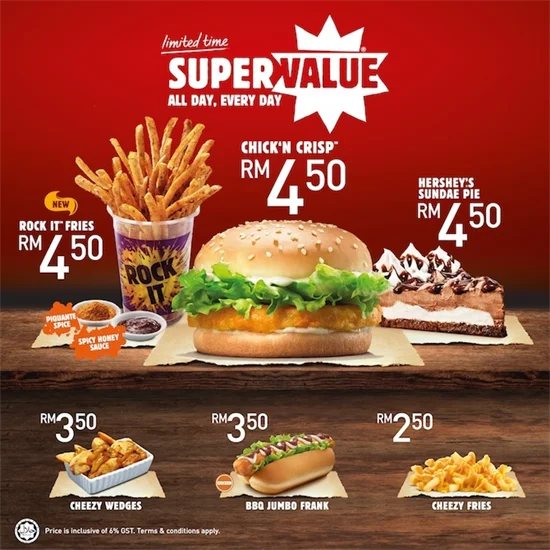
