
"I love Python Data - Python for Data Analytics"
www.pythondata.com VS www.gqak.com
2022-05-02 11:16:33
Skip to content HomeAboutContactWork With Me Market Basket Analysis with Python and Pandas Posted on December 26, 2019December 26, 2019 by Eric D. Brown, D.Sc.If you’ve ever worked with retail data, you’ll most likely have run across the need to perform some market basket analysis (also called Cross-Sell recommendations). If you aren’t sure what market basket analysis is, I’ve provided a quick overview below.What is Market Basket Analysis?In the simplest of terms, market basket analysis looks at retail sales data and determines what products are purchased together. For example, if you sell widgets and want to be able to recommend similar products and/or products that are purchased together, you can perform this type of analysis to be able to understand what products should be recommended when a user views a widget.You can think of this type of analysis as generating the following ‘rules’:If widget A, then recommend widget B, C and FIf widget L, then recommend widget X, Y and RWith these rules, you can then build our recommendation engines for your website, store and salespeople to use when selling products to customers. Market Basket Analysis requires a large amount of transaction data to work well. If you have a large amount of transactional data, you should be able to run a market basket analysis with ease. if you want to learn more about Market Basket Analysis, here’s some additional reading.In the remainder of this article, I show you how to do this type of analysis using python and pandas.Market Basket Analysis with Python and PandasThere are a few approaches that you can take for this type of analysis. You can use a pre-built library like MLxtend or you can build your own algorithm. I prefer the MLxtend library myself, but recently there’s been some memory issues using pandas and large datasets with MLxtend, so there have been times that I’ve needed to roll my own.Below, I provide an example of using MLxtend as well as an example of how to roll your own analysis.Market Basket Analysis with MLxtendFor this example, we’ll use the data set found here. This data-set contains enough data to be useful in understanding market basket analysis but isn’t too large that we can’t use MLxtend (because we can’t unstack the data, which is required to use MLxtend ).To get started, you’ll need to have pandas and MLxtend installed:pip install pandas mlxtendThen, import your libraries:import pandas as pdfrom mlxtend.frequent_patterns import apriorifrom mlxtend.frequent_patterns import association_rulesNow, lets read in the data and then drop any rows that don’t have an invoice number. Lastly, we’ll convert the `InvoiceNo` column to a string. NOTE: I downloaded the data file from here and stored it in a subdirectory named `data`.df = pd.read_excel('data/Online Retail.xlsx')df.dropna(axis=0, subset=['InvoiceNo'], inplace=True)df['InvoiceNo'] = df['InvoiceNo'].astype('str')In this data, there are some invoices that are ‘credits’ instead of ‘debits’ so we want to remove those. They are indentified with “C” in the InvoiceNo field. We can see an example of these types of invoices with the following:df[df.InvoiceNo.str.contains('C', na=False)].head()To remove these credit invoices, we can find all invoices with ‘C’ in them, and take the inverse of the results. That can be accomplished with the following line of code:df = df[~df['InvoiceNo'].str.contains('C')]Now, we are ready to start our market basket analysis. First, we’ll groupby the columns that we want to consider. For the purposes of this analysis, we’ll only look at the United Kingdom orders.market_basket = df[df['Country'] =="United Kingdom"].groupby( ['InvoiceNo', 'Description'])['Quantity']Next, we want to hot encode the data and get 1 transaction per row to prepare to run our mlxtend analysis.market_basket = market_basket.sum().unstack().reset_index().fillna(0).set_index('InvoiceNo')Let’s take a look at the output:market_basket.head()Looks like a bunch of zeros. What good is that? Well…its exactly what we want to see. We’ve encoded our data to show when a product is sold with another product. If there is a zero, that means those products haven’t sold together. Before we continue, we want to convert all of our numbers to either a `1` or a `0` (negative numbers are converted to zero, positive numbers are converted to 1). We can do this encoding step with the following function:def encode_data(datapoint): if datapoint = 1: return 1And now, we do our final encoding step:market_basket = market_basket.applymap(encode_data)Now, lets find out which items are frequently purchased together. We do this by applying the mlxtend `apriori` fuuinction to our dataset.There one thing we need to think about first. the `apriori` function requires us to provide a minimum level of ‘support’. Support is defined as the percentage of time that an itemset appears in the dataset. If you set support = 50%, you’ll only get itemsets that appear 50% of the time. I like to set support to around 5% when starting out to be able to see some data/results and then adjust from there. Setting the support level to high could lead to very few (or no) results and setting it too low could require an enormous amount of memory to process the data.In the case of this data, I originally set the `min_support` to 0.05 but didn’t receive any results, so I changed it to 0.03.itemsets = apriori(market_basket, min_support=0.03, use_colnames=True)The final step is to build your association rules using the mxltend `association_rules` function. You can set the metric that you are most interested in (either `lift` or `confidence` and set the minimum threshold for the condfidence level (called `min_threshold`). The `min_threshold` can be thought of as the level of confidence percentage that you want to return. For example, if you set `min_threshold` to 1, you will only see rules with 100% confidence. I usually set this to 0.7 to start with.rules = association_rules(itemsets, metric="lift", min_threshold=0.5)With this, we generate 16 rules for our market basket analysis.This gives us a good number of data points to look at for this analysis. Now, what does this tell us?If you look in the `antecedents` column and the `consequents` column, you’ll see names of products. Each rule tells us that the `antecedents` is sold along with the `consequents`. You can use this information to build a cross-sell recommendation system that promotes these products with each other on your website (or in person when doing in-person sales).Without knowing much more about the business that generated this data, we can’t really do much more with it. If you were using your own data, you’d be able to dig a bit deeper to find those rules with higher confidence and/or lift to help you understand the items that are sold together most often and start building strategies to promote those items (or other items if you are trying to grow sales in other areas of your business).When can you not use MLxtend?MLxtend can be used anytime you want and it is my preferred approach for market basket analysis. That said, there’s an issue (as of the date of this article) with using pandas with large datasets when performing the step of unstacking the data with this line:market_basket = market_basket.sum().unstack().reset_index().fillna(0).set_index('InvoiceNo')You can see the issue here.When you run across this issue, you’ll need to find an approach to running a market basket analysis. You can probably find ways to work around the pandas unstack problem, but what I’ve done recently is just roll my own analysis (its actually pretty simple to do). That’s what I’ll show you below.To get started, we need to import a few more libraries:from itertools import combinations, groupbyfrom collections import CounterLet’s use our original dataframe and assign it to a new df so we know we are working with a completely new data-set vs the above. We’ll use the same United Kingdom filter that we did beforedf_manual = df[df['Country'] =="United Kingdom"]Now, lets grab just the order data. For this,we’ll get the InvoiceNo and StockCode columns since all we care about is whether an item exists on an invoice. Remember, we’ve already removed the ‘credit’ invoices in the above steps so all we have are regular invoices. NOTE: There *will* be differences in the output of this approach vs MLxtend’s approach just like there will be differences in other approaches you might use for market basket analysis.orders = df_manual.set_index('InvoiceNo')['StockCode']Now that we have a pandas series of Items, Let’s calculate the item frequency and support values.statistics = orders.value_counts().to_frame("frequency")statistics['support'] = statistics / len(set(orders.index)) * 100Let’s filter out any rows of data that doesn’t have support above our min_support levelmin_support=0.03 # same value we used above.items_above_support = statistics[statistics['support'] >= min_support].indexorders_above_support = orders[orders.isin(items_above_support)]We next need to filter out orders that only had 1 items ordered on the invoice, since those items won’t provide any insight into our market basket analysis.order_counts = orders.index.value_counts()orders_over_two_index = order_counts[order_counts>=2].indexorders_over_two = orders[orders.index.isin(orders_over_two_index)]Now, let’s calculate our stats dataframe again with this new order data-set.statistics = orders_over_two.value_counts().to_frame("frequency")statistics['support'] = statistics / len(set(orders_over_two.index)) * 100Time to do the fun stuff. Calculating the itemsets / item pairs. We’ll create a function that will generate our itemsets and then send our new order dataset through the generator. Then, we calculate the frequency of each item with each other (named `frequencyAC`) as well as the support (named `supportAC`). Finally, we filter out the itemsets that are below our `min_support` leveldef itemset_generator(orders): orders = orders.reset_index().values for order_id, order_object in groupby(orders, lambda x: x[0]): item_list = [item[1] for item in order_object] for item_pair in combinations(item_list, 2): yield item_pairitemsets_gen = itemset_generator(orders_over_two)itemsets = pd.Series(Counter(itemsets_gen)).to_frame("frequencyAC")itemsets['supportAC'] = itemsets['frequencyAC'] / len(orders_over_two_index) * 100itemsets = itemsets[itemsets['supportAC'] >= min_support]Finally, we can calculate our association rules. First, let’s unstack our itemsets and create the necessary data columns for support, lift, etc.# Create table of association rules and compute relevant metricsitemsets = itemsets.reset_index().rename(columns={'level_0': 'antecedents', 'level_1': 'consequents'})itemsets = (itemsets .merge(statistics.rename(columns={'freq': 'freqA', 'support': 'antecedent support'}), left_on='antecedents', right_index=True) .merge(statistics.rename(columns={'freq': 'freqC', 'support': 'consequents support'}), left_on='consequents', right_index=True))itemsets['confidenceAtoC'] = itemsets['supportAC'] / itemsets['antecedent support']itemsets['confidenceCtoA'] = itemsets['supportAC'] / itemsets['consequents support']itemsets['lift'] = itemsets['supportAC'] / (itemsets['antecedent support'] * itemsets['consequents support'])itemsets=itemsets[['antecedents', 'consequents','antecedent support', 'consequents support', 'confidenceAtoC','lift']]Finally, let’s look at our final rules. We want to look at only those items that have confidence > 0.5.rules = itemsetsrules_over_50 = rules[(rules.confidenceAtoC >0.50)]rules_over_50.set_index('antecedents',inplace=True)rules_over_50.reset_index(inplace=True)rules_over_50=rules_over_50.sort_values('lift', ascending=False)Looking at the rules_over_50 data, we see our final set of rules using our ‘roll your own’ approach.These rules are going to be a bit different than what we get with MLxtend, but that’s OK as it gives us another set of data to look at – and the only set of data to look at when your data is too large to use MLxtend. One extension to this approach would be to add in a step to replace the stockcode numbers with the item descriptions. I’ll leave it to you to do that work. Posted in data analytics, pythonTagged apriori, cross-sell, market basket analysis, pandas, pythonComparing Machine Learning Methods Posted on March 20, 2019December 25, 2019 by Eric D. Brown, D.Sc.When working with data and modeling, its sometimes hard to determine what model you should use for a particular modeling project. A quick way to find an algorithm that might work better than others is to run through an algorithm comparison loop to see how various models work against your data. In this post, I’ll be comparing machine learning methods using a few different sklearn algorithms. As always, you can find a jupyter notebook for this article on my github here and find other articles on this topic here.I’ve used Jason Brownlee’s article from 2016 as the basis for this article…I wanted to expand a bit on what he did as well as use a different dataset. In this article, we’ll be using the Indian Liver Disease dataset (found here).From the dataset page:This data set contains 416 liver patient records and 167 non liver patient records collected from North East of Andhra Pradesh, India. The “Dataset” column is a class label used to divide groups into liver patient (liver disease) or not (no disease). This data set contains 441 male patient records and 142 female patient records.Let’s get started by setting up our imports that we’ll use.import pandas as pdimport matplotlib.pyplot as pltplt.rcParams["figure.figsize"] = (20,10)from sklearn import model_selectionfrom sklearn.linear_model import LogisticRegressionfrom sklearn.svm import SVCfrom sklearn.neighbors import KNeighborsClassifierfrom sklearn.tree import DecisionTreeClassifierfrom sklearn.naive_bayes import GaussianNBfrom sklearn.discriminant_analysis import LinearDiscriminantAnalysisNext, we’ll read in the data from the CSV file located in the local directory.#read in the datadata = pd.read_csv('indian_liver_patient.csv')If you do a head() of the dataframe, you’ll get a good feeling for the dataset.We’ll use all columns except Gender for this tutorial. We could use gender by converting the gender to a numeric value (e.g., 0 for Male, 1 for Female) but for the purposes of this post, we’ll just skip this column.data_to_use = datadel data_to_use['Gender']data_to_use.dropna(inplace=True)The ‘Dataset’ column is the value we are trying to predict…whether the user has liver disease or not so we’ll that as our “Y” and the other columns for our “X” array.values = data_to_use.valuesY = values[:,9]X = values[:,0:9]Before we run our machine learning models, we need to set a random number to use to seed them. This can be any random number that you’d like it to be. Some people like to use a random number generator but for the purposes of this, I’ll just set it to 12 (it could just as easily be 1 or 3 or 1023 or any other number).random_seed = 12Now we need to set up our models that we’ll be testing out. We’ll set up a list of the models and give them each a name. Additionally, I’m going to set up the blank arrays/lists for the outcomes and the names of the models to use for comparison.outcome = []model_names = []models = [('LogReg', LogisticRegression()), ('SVM', SVC()), ('DecTree', DecisionTreeClassifier()), ('KNN', KNeighborsClassifier()), ('LinDisc', LinearDiscriminantAnalysis()), ('GaussianNB', GaussianNB())]We are going to use a k-fold validation to evaluate each algorithm and will run through each model with a for loop, running the analysis and then storing the outcomes into the lists we created above. We’ll use a 10-fold cross validation.for model_name, model in models: k_fold_validation = model_selection.KFold(n_splits=10, random_state=random_seed) results = model_selection.cross_val_score(model, X, Y, cv=k_fold_validation, scoring='accuracy') outcome.append(results) model_names.append(model_name) output_message = "%s| Mean=%f STD=%f" % (model_name, results.mean(), results.std()) print(output_message)The output from this loop is:LogReg| Mean=0.718633 STD=0.058744SVM| Mean=0.715124 STD=0.058962DecTree| Mean=0.637568 STD=0.108805KNN| Mean=0.651301 STD=0.079872LinDisc| Mean=0.716878 STD=0.050734GaussianNB| Mean=0.554719 STD=0.081961From the above, it looks like the Logistic Regression, Support Vector Machine and Linear Discrimination Analysis methods are providing the best results (based on the ‘mean’ values). Taking Jason’s lead, we can take a look at a box plot to see what the accuracy is for each cross validation fold, we can see just how good each does relative to each other and their means.fig = plt.figure()fig.suptitle('Machine Learning Model Comparison')ax = fig.add_subplot(111)plt.boxplot(outcome)ax.set_xticklabels(model_names)plt.show()From the box plot, when it is easy to see the three mentioned machine learning methods (Logistic Regression, Support Vector Machine and Linear Discrimination Analysis) are providing better accuracies. From this outcome, we can then take this data and start working with these three models to see how we might be able to optimize the modeling process to see if one model works a bit better than others. Posted in machine learningTagged data science, machine learning, python4 Comments on Comparing Machine Learning MethodsQuick Tip – Speed up Pandas using Modin Posted on February 7, 2019February 7, 2019 by Eric D. Brown, D.Sc.I ran across a neat little library called Modin recently that claims to run pandas faster. The one line sentence that they use to describe the project is:Speed up your Pandas workflows by changing a single line of codeInteresting…and important if true.Using modin only requires importing modin instead of pandas and thats it…no other changes required to your existing code.One caveat – modin currently uses pandas 0.20.3 (at least it installs pandas 0.20. when modin is installed with pip install modin). If you’re using the latest version of pandas and need functionality that doesn’t exist in previous versions, you might need to wait on checking out modin – or play around with trying to get it to work with the latest version of pandas (I haven’t done that yet).To install modin:pip install modinTo use modin:import modin.pandas as pdThat’s it. Rather than import pandas as pd you import modin.pandas as pd and you get all the advantages of additional speed. A Read CSV Benchmark provided by ModinAccording to the documentation, modin takes advantage of multi-cores on modern machines, which pandas does not do. From their website:In pandas, you are only able to use one core at a time when you are doing computation of any kind. With Modin, you are able to use all of the CPU cores on your machine. Even in read_csv, we see large gains by efficiently distributing the work across your entire machine.Let’s give is a shot and see how it works.For this test, I’m going to try out their read_csv method since its something they highlight. For this test, I have a 105 MB csv file. Lets time both pandas and modin and see how things work.We’ll start with pandas.from timeit import default_timer as timerimport pandas as pd# run 25 iterations of read_csv to get an averagetime = []for i in range (0, 25): start = timer() df = pd.read_csv('OSMV-20190206.csv') end = timer() time.append((end - start)) # print out the average time taken # I *think* I got this little trick from # from https://stackoverflow.com/a/9039992/2887031print reduce(lambda x, y: x + y, time) / len(time)With pandas, it seems to take – on average – 1.26 seconds to read a 105MB csv file.Now, lets take a look at modin.Before continuing, I should share that I had to do a couple extra steps to get modin to work beyond just pip install modin. I had to install typing and dask as well.pip install "modin[dask]"pip install typingUsing the exact same code as above (except one minor change to import modin — import modin.pandas as pd.from timeit import default_timer as timerimport modin.pandas as pd# run 25 iterations of read_csv to get an averagetime = []for i in range (0, 25): start = timer() df = pd.read_csv('OSMV-20190206.csv') end = timer() time.append((end - start)) # print out the average time taken # I *think* I got this little trick from # from https://stackoverflow.com/a/9039992/2887031print reduce(lambda x, y: x + y, time) / len(time)With modin, it seems to take – on average – 0.96 seconds to read a 105MB csv file.Using modin – in this example – I was able to shave off 0.3 seconds from the average read time for reading in that 105MB csv file. That may not seem like a lot of time, but it is a savings of around 27%. Just imagine if you’ve got 5000 csv files to read in that are of similar size, that’s a savings of 1500 seconds on average…that’s 25 minutes of time saved in just reading files.Modin uses Ray to speed pandas up, so there could be even more savings if you get in and play around with some of the settings of Ray.I’ll be looking at modin more in the future to use in some of my projects to help gain some efficiencies. Take a look at it and let me know what you think. Posted in pandas, pythonTagged efficiency, modin, pandas, python, time savings14 Comments on Quick Tip – Speed up Pandas using ModinPosts navigationOlder postsSign up for our newsletterRecent Posts Market Basket Analysis with Python and Pandas Comparing Machine Learning Methods Quick Tip – Speed up Pandas using Modin Stationary Data Tests for Time Series Forecasting Forecasting Time Series Data using AutoregressionCategoriesbook reveiwClouddata analyticsForecastinggetting startedlinuxmacmachine learningOS Xpandasprophetpythonquick tipsSocial Networksstock markettext analyticstime seriesvagrantvisualizationWindowsAbout this siteBig data. Data analytics. Data science. Data processing. Predictive Analytics.Regardless of what needs to be done or what you call the activity, the first thing you need to now is “how” to analyze data. You also need to have a tool set for analyzing data.If you work for a large company, you may have a full blown big data suite of tools and systems to assist in your analytics work. Otherwise, you may have nothing but excel and open source tools to perform your analytics activities.This post and this site is for those of you who don’t have the ‘big data’ systems and suites available to you. On this site, we’ll be talking about using python for data analytics. I started this blog as a place for me write about working with python for my various data analytics projects.Learn more…Copyright 2017 All Right Revised.Theme Designed By TallyThemes | Powered by WordPress Close this module If you'd like to receive updates when new posts are published, signup for my mailing list. I won't sell or share your email. @media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-slidein-content .hustle-optin {max-width: 800px;}}.hustle-ui.module_id_1 .hustle-slidein-content {-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0.2);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0.2);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0.2);}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-slidein-content {-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0.2);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0.2);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0.2);}} .hustle-ui.module_id_1 .hustle-layout .hustle-layout-body {margin: 0px 0px 0px 0px;padding: 0px 0px 0px 0px;border-width: 0px 0px 0px 0px;border-style: solid;border-color: rgba(218,218,218,1);border-radius: 0px 0px 0px 0px;overflow: hidden;background-color: #38454e;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-layout-body {margin: 0px 0px 0px 0px;padding: 0px 0px 0px 0px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;}} .hustle-ui.module_id_1 .hustle-layout .hustle-layout-content {padding: 0px 0px 0px 0px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;border-color: rgba(0,0,0,0);background-color: rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-layout-content {padding: 0px 0px 0px 0px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}} .hustle-ui.module_id_1 .hustle-layout .hustle-content {margin: 0px 0px 0px 0px;padding: 0 10px 0 10px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;border-color: rgba(0,0,0,0);background-color: rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}.hustle-ui.module_id_1 .hustle-layout .hustle-content .hustle-content-wrap {padding: 10px 0 10px 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-content {margin: 0px 0px 0px 0px;padding: 0 20px 0 20px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-content .hustle-content-wrap {padding: 20px 0 20px 0;}} .hustle-ui.module_id_1 .hustle-layout .hustle-group-content {margin: 0px 0px 0px 0px;padding: 0px 0px 0px 0px;border-color: rgba(0,0,0,0);border-width: 0px 0px 0px 0px;border-style: solid;color: #adb5b7;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content b,.hustle-ui.module_id_1 .hustle-layout .hustle-group-content strong {font-weight: bold;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content a,.hustle-ui.module_id_1 .hustle-layout .hustle-group-content a:visited {color: #38c5b5;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content a:hover {color: #49e2d1;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content a:focus,.hustle-ui.module_id_1 .hustle-layout .hustle-group-content a:active {color: #49e2d1;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content {margin: 0px 0px 0px 0px;padding: 0px 0px 0px 0px;border-width: 0px 0px 0px 0px;border-style: solid;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content {color: #adb5b7;font-size: 14px;line-height: 1.45em;font-family: Open Sans;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content {font-size: 14px;line-height: 1.45em;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content p:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font: normal 14px/1.45em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content p:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content p:not([class*="forminator-"]) {margin: 0 0 10px;font: normal 14px/1.45em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content p:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h1:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font: 700 28px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h1:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h1:not([class*="forminator-"]) {margin: 0 0 10px;font: 700 28px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h1:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h2:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font-size: 22px;line-height: 1.4em;font-weight: 700;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h2:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h2:not([class*="forminator-"]) {margin: 0 0 10px;font-size: 22px;line-height: 1.4em;font-weight: 700;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h2:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h3:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font: 700 18px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h3:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h3:not([class*="forminator-"]) {margin: 0 0 10px;font: 700 18px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h3:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h4:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font: 700 16px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h4:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h4:not([class*="forminator-"]) {margin: 0 0 10px;font: 700 16px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h4:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h5:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font: 700 14px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h5:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h5:not([class*="forminator-"]) {margin: 0 0 10px;font: 700 14px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h5:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h6:not([class*="forminator-"]) {margin: 0 0 10px;color: #adb5b7;font: 700 12px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: uppercase;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content h6:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h6:not([class*="forminator-"]) {margin: 0 0 10px;font: 700 12px/1.4em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: uppercase;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content h6:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ol:not([class*="forminator-"]),.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ul:not([class*="forminator-"]) {margin: 0 0 10px;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ol:not([class*="forminator-"]):last-child,.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ul:not([class*="forminator-"]):last-child {margin-bottom: 0;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content li:not([class*="forminator-"]) {margin: 0 0 5px;color: #adb5b7;font: normal 14px/1.45em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content li:not([class*="forminator-"]):last-child {margin-bottom: 0;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ol:not([class*="forminator-"]) li:before {color: #ADB5B7}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ul:not([class*="forminator-"]) li:before {background-color: #ADB5B7}@media screen and (min-width: 783px) {.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ol:not([class*="forminator-"]),.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ul:not([class*="forminator-"]) {margin: 0 0 20px;}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ol:not([class*="forminator-"]):last-child,.hustle-ui.module_id_1 .hustle-layout .hustle-group-content ul:not([class*="forminator-"]):last-child {margin: 0;}}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content li:not([class*="forminator-"]) {margin: 0 0 5px;font: normal 14px/1.45em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-group-content li:not([class*="forminator-"]):last-child {margin-bottom: 0;}}.hustle-ui.module_id_1 .hustle-layout .hustle-group-content blockquote {margin-right: 0;margin-left: 0;}.hustle-ui.module_id_1 button.hustle-button-close {color: #38c5b5;}.hustle-ui.module_id_1 button.hustle-button-close:hover {color: #49e2d1;}.hustle-ui.module_id_1 button.hustle-button-close:focus {color: #49e2d1;} .hustle-ui.module_id_1 .hustle-layout .hustle-layout-form {margin: 0px 0px 0px 0px;padding: 10px 10px 10px 10px;border-width: 0px 0px 0px 0px;border-style: solid;border-color: rgba(0,0,0,0);border-radius: 0px 0px 0px 0px;background-color: #5d7380;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-layout-form {margin: 0px 0px 0px 0px;padding: 20px 20px 20px 20px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;}}.hustle-ui.module_id_1 .hustle-form .hustle-form-fields {display: block;}.hustle-ui.module_id_1 .hustle-form .hustle-form-fields .hustle-field {margin-bottom: 1px;}.hustle-ui.module_id_1 .hustle-form .hustle-form-fields .hustle-button {width: 100%;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-form-fields {display: -webkit-box;display: -ms-flex;display: flex;-ms-flex-wrap: wrap;flex-wrap: wrap;-webkit-box-align: center;-ms-flex-align: center;align-items: center;margin-top: -0.5px;margin-bottom: -0.5px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-form-fields .hustle-field {min-width: 100px;-webkit-box-flex: 1;-ms-flex: 1;flex: 1;margin-top: 0.5px;margin-right: 1px;margin-bottom: 0.5px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-form-fields .hustle-button {width: auto;-webkit-box-flex: 0;-ms-flex: 0 0 auto;flex: 0 0 auto;margin-top: 0.5px;margin-bottom: 0.5px;}} .hustle-ui.module_id_1 .hustle-field .hustle-input {margin: 0;padding: 9px 10px 9px 10px;padding-left: calc(10px + 25px);border-width: 1px 1px 1px 1px;border-style: solid;border-color: rgba(218,218,218,1);border-radius: 0px 0px 0px 0px;background-color: #fdfdfd;box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);color: #363b3f;font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}.hustle-ui.module_id_1 .hustle-field .hustle-input:hover {border-color: rgba(218,218,218,1);background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-field .hustle-input:focus {border-color: rgba(218,218,218,1);background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-field-error.hustle-field .hustle-input {border-color: #D43858 !important;background-color: #fdfdfd !important;}.hustle-ui.module_id_1 .hustle-field .hustle-input + .hustle-input-label [class*="hustle-icon-"] {color: #adb5b7;}.hustle-ui.module_id_1 .hustle-field .hustle-input:hover + .hustle-input-label [class*="hustle-icon-"] {color: #adb5b7;}.hustle-ui.module_id_1 .hustle-field .hustle-input:focus + .hustle-input-label [class*="hustle-icon-"] {color: #adb5b7;}.hustle-ui.module_id_1 .hustle-field-error.hustle-field .hustle-input + .hustle-input-label [class*="hustle-icon-"] {color: #D43858;}.hustle-ui.module_id_1 .hustle-field .hustle-input + .hustle-input-label {padding: 9px 10px 9px 10px;border-width: 1px 1px 1px 1px;border-style: solid;border-color: transparent;color: #adb5b7;font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-field .hustle-input {padding: 9px 10px 9px 10px;padding-left: calc(10px + 25px);border-width: 1px 1px 1px 1px;border-style: solid;border-radius: 0px 0px 0px 0px;box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-field .hustle-input + .hustle-input-label {padding: 9px 10px 9px 10px;border-width: 1px 1px 1px 1px;font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}} .hustle-ui.module_id_1 .hustle-select2 + .select2 {box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}.hustle-ui.module_id_1 .hustle-select2 + .select2 .select2-selection--single {margin: 0;padding: 0 10px 0 10px;border-width: 1px 1px 1px 1px;border-style: solid;border-color: #FFFFFF;border-radius: 0px 0px 0px 0px;background-color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-select2 + .select2 .select2-selection--single .select2-selection__rendered {padding: 9px 0 9px 0;color: #5D7380;font: normal 13px/18px Open Sans;font-style: normal;}.hustle-ui.module_id_1 .hustle-select2 + .select2 .select2-selection--single .select2-selection__rendered .select2-selection__placeholder {color: #AAAAAA;}.hustle-ui.module_id_1 .hustle-select2 + .select2:hover .select2-selection--single {border-color: #FFFFFF;background-color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-select2 + .select2.select2-container--open .select2-selection--single {border-color: #FFFFFF;background-color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-select2.hustle-field-error + .select2 .select2-selection--single {border-color: #FFFFFF !important;background-color: #FFFFFF !important;}.hustle-ui.module_id_1 .hustle-select2 + .select2 + .hustle-input-label {color: #AAAAAA;font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}.hustle-ui.module_id_1 .hustle-select2 + .select2 .select2-selection--single .select2-selection__arrow {color: #38C5B5;}.hustle-ui.module_id_1 .hustle-select2 + .select2:hover .select2-selection--single .select2-selection__arrow {color: #49E2D1;}.hustle-ui.module_id_1 .hustle-select2 + .select2.select2-container--open .select2-selection--single .select2-selection__arrow {color: #49E2D1;}.hustle-ui.module_id_1 .hustle-select2.hustle-field-error + .select2 .select2-selection--single .select2-selection__arrow {color: #D43858 !important;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-select2 + .select2 {box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-select2 + .select2 .select2-selection--single {padding: 0 10px 0 10px;border-width: 1px 1px 1px 1px;border-style: solid;border-radius: 0px 0px 0px 0px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-select2 + .select2 .select2-selection--single .select2-selection__rendered {padding: 9px 0 9px 0;font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-select2 + .select2 + .hustle-input-label {font: normal 13px/18px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-align: left;}}.hustle-module-1.hustle-dropdown {background-color: #FFFFFF;}.hustle-module-1.hustle-dropdown .select2-results .select2-results__options .select2-results__option {color: #5D7380;background-color: transparent;}.hustle-module-1.hustle-dropdown .select2-results .select2-results__options .select2-results__option.select2-results__option--highlighted {color: #FFFFFF;background-color: #ADB5B7;}.hustle-module-1.hustle-dropdown .select2-results .select2-results__options .select2-results__option[aria-selected="true"] {color: #FFFFFF;background-color: #38C5B5;}.hustle-ui.module_id_1 .hustle-timepicker .ui-timepicker {background-color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-timepicker .ui-timepicker .ui-timepicker-viewport a {color: #5D7380;background-color: transparent;}.hustle-ui.module_id_1 .hustle-timepicker .ui-timepicker .ui-timepicker-viewport a:hover,.hustle-ui.module_id_1 .hustle-timepicker .ui-timepicker .ui-timepicker-viewport a:focus {color: #FFFFFF;background-color: #ADB5B7;} .hustle-ui.module_id_1 .hustle-form .hustle-radio span[aria-hidden] {border-width: 0px 0px 0px 0px;border-style: solid;border-color: #FFFFFF;background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-form .hustle-radio span:not([aria-hidden]) {color: #adb5b7;font: normal 12px/20px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}.hustle-ui.module_id_1 .hustle-form .hustle-radio input:checked + span[aria-hidden] {border-color: #FFFFFF;background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-form .hustle-radio input:checked + span[aria-hidden]:before {background-color: #38c5b5;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-radio span[aria-hidden] {border-width: 0px 0px 0px 0px;border-style: solid;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-radio span:not([aria-hidden]) {font: normal 12px/20px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}} .hustle-ui.module_id_1 .hustle-form .hustle-checkbox:not(.hustle-gdpr) span[aria-hidden] {border-width: 0px 0px 0px 0px;border-style: solid;border-color: #FFFFFF;border-radius: 0px 0px 0px 0px;background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-form .hustle-checkbox:not(.hustle-gdpr) span:not([aria-hidden]) {color: #adb5b7;font: normal 12px/20px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}.hustle-ui.module_id_1 .hustle-form .hustle-checkbox:not(.hustle-gdpr) input:checked + span[aria-hidden] {border-color: #FFFFFF;background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-form .hustle-checkbox:not(.hustle-gdpr) input:checked + span[aria-hidden]:before {color: #38c5b5;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-checkbox:not(.hustle-gdpr) span[aria-hidden] {border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-checkbox:not(.hustle-gdpr) span:not([aria-hidden]) {font: normal 12px/20px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}}.hustle-module-1.hustle-calendar:before {background-color: #FFFFFF;}.hustle-module-1.hustle-calendar .ui-datepicker-header .ui-datepicker-title {color: #35414A;}.hustle-module-1.hustle-calendar .ui-datepicker-header .ui-corner-all,.hustle-module-1.hustle-calendar .ui-datepicker-header .ui-corner-all:visited {color: #5D7380;}.hustle-module-1.hustle-calendar .ui-datepicker-header .ui-corner-all:hover {color: #5D7380;}.hustle-module-1.hustle-calendar .ui-datepicker-header .ui-corner-all:focus,.hustle-module-1.hustle-calendar .ui-datepicker-header .ui-corner-all:active {color: #5D7380;}.hustle-module-1.hustle-calendar .ui-datepicker-calendar thead th {color: #35414A;}.hustle-module-1.hustle-calendar .ui-datepicker-calendar tbody tr td a,.hustle-module-1.hustle-calendar .ui-datepicker-calendar tbody tr td a:visited {background-color: #FFFFFF;color: #5D7380;}.hustle-module-1.hustle-calendar .ui-datepicker-calendar tbody tr td a:hover {background-color: #38C5B5;color: #FFFFFF;}.hustle-module-1.hustle-calendar .ui-datepicker-calendar tbody tr td a:focus,.hustle-module-1.hustle-calendar .ui-datepicker-calendar tbody tr td a:active {background-color: #38C5B5;color: #FFFFFF;} .hustle-ui.module_id_1 .hustle-form button.hustle-button-submit {padding: 2px 16px 2px 16px;border-width: 0px 0px 0px 0px;border-style: solid;border-color: #dadada;border-radius: 0px 0px 0px 0px;background-color: #38c5b5;-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);color: #fdfdfd;font: bold 13px/32px Open Sans;font-style: normal;letter-spacing: 0.5px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-form button.hustle-button-submit:hover {border-color: #dadada;background-color: #49e2d1;color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-form button.hustle-button-submit:focus {border-color: #dadada;background-color: #49e2d1;color: #fdfdfd;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form button.hustle-button-submit {padding: 2px 16px 2px 16px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);font: bold 13px/32px Open Sans;font-style: normal;letter-spacing: 0.5px;text-transform: none;text-decoration: none;}} .hustle-ui.module_id_1 .hustle-form .hustle-form-options {margin: 10px 0px 0px 0px;padding: 20px 20px 20px 20px;border-width: 0px 0px 0px 0px;border-style: solid;border-color: rgba(0,0,0,0);border-radius: 0px 0px 0px 0px;background-color: #35414A;-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-form-options {margin: 10px 0px 0px 0px;padding: 20px 20px 20px 20px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}} .hustle-ui.module_id_1 .hustle-form .hustle-form-options .hustle-group-title {display: block;margin: 0 0 20px;padding: 0;border: 0;color: #fdfdfd;font: bold 13px/22px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-form .hustle-form-options .hustle-group-title {font: bold 13px/22px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}} .hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr {margin: 10px 0px 0px 0px;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span[aria-hidden] {border-width: 2px 2px 2px 2px;border-style: solid;border-color: #dadada;border-radius: 0px 0px 0px 0px;background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span[aria-hidden]:before {color: #38C5B5;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span:not([aria-hidden]) {color: #ADB5B7;font: normal 12px/1.7em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span:not([aria-hidden]) a {color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span:not([aria-hidden]) a:hover {color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span:not([aria-hidden]) a:focus {color: #FFFFFF;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr input:checked + span[aria-hidden] {border-color: #dadada;background-color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr.hustle-field-error + span[aria-hidden] {border-color: #D43858 !important;background-color: #FFFFFF !important;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr {margin: 10px 0px 0px 0px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span[aria-hidden] {border-width: 2px 2px 2px 2px;border-style: solid;border-radius: 0px 0px 0px 0px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout-form .hustle-checkbox.hustle-gdpr span:not([aria-hidden]) {font: normal 12px/1.7em Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}} .hustle-ui.module_id_1 .hustle-layout .hustle-error-message {margin: 20px 0px 0px 0px;background-color: #ea6464;box-shadow: inset 4px 0 0 0 #D43858;-moz-box-shadow: inset 4px 0 0 0 #D43858;-webkit-box-shadow: inset 4px 0 0 0 #D43858;}.hustle-ui.module_id_1 .hustle-layout .hustle-error-message p {color: #f1f1f1;font: normal 12px/20px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-error-message {margin: 20px 0px 0px 0px;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-layout .hustle-error-message p {font: normal 12px/20px Open Sans;font-style: normal;letter-spacing: 0px;text-transform: none;text-decoration: none;text-align: left;}} .hustle-ui.module_id_1 .hustle-success {padding: 40px 40px 40px 40px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;border-color: rgba(0,0,0,0);background-color: #38454E;box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);color: #fdfdfd;}.hustle-ui.module_id_1 .hustle-success [class*="hustle-icon-"] {color: #37c6b5;}.hustle-ui.module_id_1 .hustle-success a,.hustle-ui.module_id_1 .hustle-success a:visited {color: #38c5b5;}.hustle-ui.module_id_1 .hustle-success a:hover {color: #49e2d1;}.hustle-ui.module_id_1 .hustle-success a:focus,.hustle-ui.module_id_1 .hustle-success a:active {color: #49e2d1;}.hustle-ui.module_id_1 .hustle-success-content b,.hustle-ui.module_id_1 .hustle-success-content strong {font-weight: bold;}.hustle-ui.module_id_1 .hustle-success-content blockquote {margin-right: 0;margin-left: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success {padding: 40px 40px 40px 40px;border-width: 0px 0px 0px 0px;border-style: solid;border-radius: 0px 0px 0px 0px;box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-moz-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);-webkit-box-shadow: 0px 0px 0px 0px rgba(0,0,0,0);}}.hustle-ui.module_id_1 .hustle-success-content {color: #fdfdfd;font-size: 14px;line-height: 1.45em;font-family: Open Sans;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content {font-size: 14px;line-height: 1.45em;}}.hustle-ui.module_id_1 .hustle-success-content p:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: normal 14px/1.45em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content p:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content p:not([class*="forminator-"]) {margin-bottom: 20px;font: normal 14px/1.45em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content p:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content h1:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: 700 28px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content h1:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h1:not([class*="forminator-"]) {margin-bottom: 20px;font: 700 28px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h1:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content h2:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: 700 22px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content h2:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h2:not([class*="forminator-"]) {margin-bottom: 20px;font: 700 22px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h2:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content h3:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: 700 18px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content h3:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h3:not([class*="forminator-"]) {margin-bottom: 20px;font: 700 18px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h3:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content h4:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: 700 16px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content h4:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h4:not([class*="forminator-"]) {margin-bottom: 20px;font: 700 16px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h4:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content h5:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: 700 14px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content h5:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h5:not([class*="forminator-"]) {margin-bottom: 20px;font: 700 14px/1.4em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h5:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content h6:not([class*="forminator-"]) {margin: 0 0 10px;color: #fdfdfd;font: 700 12px/1.4em Open Sans;letter-spacing: 0px;text-transform: uppercase;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content h6:not([class*="forminator-"]):last-child {margin-bottom: 0;}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h6:not([class*="forminator-"]) {margin-bottom: 20px;font: 700 12px/1.4em Open Sans;letter-spacing: 0px;text-transform: uppercase;text-decoration: none;}.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content h6:not([class*="forminator-"]):last-child {margin-bottom: 0}}.hustle-ui.module_id_1 .hustle-success-content ol:not([class*="forminator-"]),.hustle-ui.module_id_1 .hustle-success-content ul:not([class*="forminator-"]) {margin: 0 0 10px;}.hustle-ui.module_id_1 .hustle-success-content ol:not([class*="forminator-"]):last-child,.hustle-ui.module_id_1 .hustle-success-content ul:not([class*="forminator-"]):last-child {margin-bottom: 0;}.hustle-ui.module_id_1 .hustle-success-content li:not([class*="forminator-"]) {margin: 0 0 5px;color: #fdfdfd;font: normal 14px/1.45em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}.hustle-ui.module_id_1 .hustle-success-content li:not([class*="forminator-"]):last-child {margin-bottom: 0;}.hustle-ui.module_id_1 .hustle-success-content ol:not([class*="forminator-"]) li:before {color: #ADB5B7}.hustle-ui.module_id_1 .hustle-success-content ul:not([class*="forminator-"]) li:before {color: #ADB5B7}@media screen and (min-width: 783px) {.hustle-ui:not(.hustle-size--small).module_id_1 .hustle-success-content li:not([class*="forminator-"]) {font: normal 14px/1.45em Open Sans;letter-spacing: 0px;text-transform: none;text-decoration: none;}}@media screen and (min-width: 783px) {.hustle-ui.module_id_1 .hustle-success-content ol:not([class*="forminator-"]),.hustle-ui.module_id_1 .hustle-success-content ul:not([class*="forminator-"]) {margin: 0 0 20px;}.hustle-ui.module_id_1 .hustle-success-content ol:not([class*="forminator-"]):last-child,.hustle-ui.module_id_1 .hustle-success-content ul:not([class*="forminator-"]):last-child {margin-bottom: 0;}} .hustle-ui.module_id_1 .hustle-layout .hustle-group-content blockquote {border-left-color: #38C5B5;}

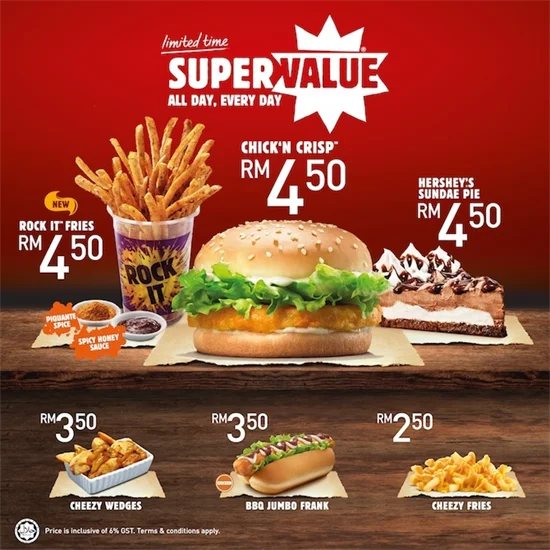
